Classes Aninhadas
Character.Subset
Character.Subset – Instâncias desta classe representam subconjuntos específicos do conjunto de caracteres Unicode.
Fonte do código: https://www.geeksforgeeks.org/java-lang-character-subset-class-java/
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
import java.lang.*; public class CharacterSubsetDemo extends Character.Subset { CharacterSubsetDemo(String s) { // Use of super keyword : // Invokes immediate parent class constructor. super(s); } public static void main(String[] args) { // Initializing two Subsets. CharacterSubsetDemo a = new CharacterSubsetDemo("geeks"); CharacterSubsetDemo b = new CharacterSubsetDemo("for"); // use of equals() : boolean check2 = a.equals(a); System.out.println("Is a equals a ? : " + check2); check2 = b.equals(a); System.out.println("Is b equals a ? : " + check2); System.out.println(); // Use of hashCode() : int check1 = a.hashCode(); System.out.println("hashCode " + a + " : " + check1); check1 = b.hashCode(); System.out.println("hashCode " + b + " : " + check1); System.out.println(); // Use of toString() : System.out.println("a : " + a.toString()); System.out.println("b : " + b.toString()); } } |
Character.UnicodeBlock
Character.UnicodeBlock – Uma família de subconjuntos de caracteres que representam os blocos de caracteres na especificação Unicode.
Fonte do código: https://stackoverflow.com/questions/1499804/how-can-i-detect-japanese-text-in-a-java-string
1 2 3 4 5 6 7 8 9 10 11 12 13 |
private boolean isCharCJK(final char c) { if ((Character.UnicodeBlock.of(c) == Character.UnicodeBlock.CJK_UNIFIED_IDEOGRAPHS) || (Character.UnicodeBlock.of(c) == Character.UnicodeBlock.CJK_UNIFIED_IDEOGRAPHS_EXTENSION_A) || (Character.UnicodeBlock.of(c) == Character.UnicodeBlock.CJK_UNIFIED_IDEOGRAPHS_EXTENSION_B) || (Character.UnicodeBlock.of(c) == Character.UnicodeBlock.CJK_COMPATIBILITY_FORMS) || (Character.UnicodeBlock.of(c) == Character.UnicodeBlock.CJK_COMPATIBILITY_IDEOGRAPHS) || (Character.UnicodeBlock.of(c) == Character.UnicodeBlock.CJK_RADICALS_SUPPLEMENT) || (Character.UnicodeBlock.of(c) == Character.UnicodeBlock.CJK_SYMBOLS_AND_PUNCTUATION) || (Character.UnicodeBlock.of(c) == Character.UnicodeBlock.ENCLOSED_CJK_LETTERS_AND_MONTHS)) { return true; } return false; } |
Character.UnicodeScript
Character.UnicodeScript – Uma família de subconjuntos de caracteres que representam os scripts de caracteres definidos no Anexo # 24 do Padrão Unicode: Nomes de Scripts.
Campos
BYTES
BYTES – O número de bytes usados para representar um valor de char no formato binário não assinado.
public static final int BYTES – O número de bytes usados para representar um valor de char no formato binário não assinado.
Esse campo existe desde a versão 1.8.
Veja também:
COMBINING_SPACING_MARK
COMBINING_SPACING_MARK – Categoria geral “Mc” na especificação Unicode.
public static final byte COMBINING_SPACING_MARK – Categoria geral “Mc” na especificação Unicode.
Esse campo existe desde a versão 1.1.
Veja também:
Fonte do código: https://stackoverflow.com/questions/29110887/detect-any-combining-character-in-java
1 2 3 4 |
String codePointStr = new String(new byte[]{(byte) 0xe1, (byte) 0x9f, (byte) 0x80}, "UTF-8"); // unicode 17c0 System.out.println(codePointStr.matches("\\p{Mc}")); System.out.println( Character.COMBINING_SPACING_MARK == Character.getType(codePointStr.codePointAt(0))); |
CONNECTOR_PUNCTUATION
CONNECTOR_PUNCTUATION – Categoria geral “Pc” na especificação Unicode.
public static final byte CONNECTOR_PUNCTUATION – Categoria geral “Pc” na especificação Unicode.
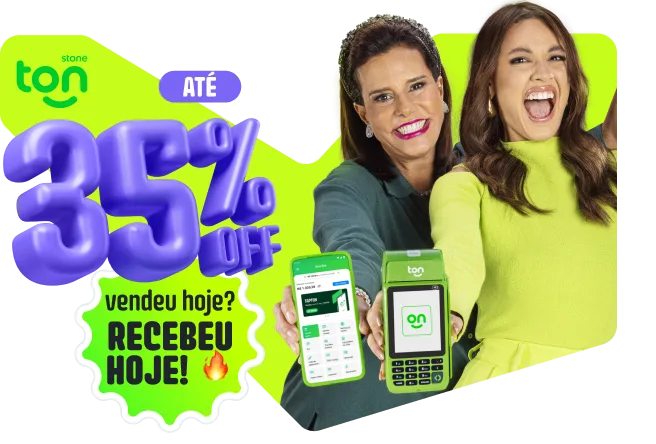
Esse método existe desde a versão 1.1.
Veja também:
CONTROL
CONTROL – Categoria geral “Cc” na especificação Unicode.
public static final byte CONTROL – Categoria geral “Cc” na especificação Unicode.
Esse método existe desde a versão 1.1.
Veja também:
CURRENCY_SYMBOL
CURRENCY_SYMBOL – Categoria geral “Sc” na especificação Unicode.
public static final byte CURRENCY_SYMBOL – Categoria geral “Sc” na especificação Unicode.
Esse método existe desde a versão 1.1.
Veja também:
Fonte do código: http://www.java2s.com/Tutorials/Java/java.lang/Character/Java_Character_CURRENCY_SYMBOL.htm
1 2 3 4 5 6 7 8 9 10 |
public class Main{ public static void main(String[] args) { for(char ch = Character.MIN_VALUE;ch<Character.MAX_VALUE;ch++){ if(Character.CURRENCY_SYMBOL == Character.getType(ch)){ String s = String.format ("\\u%04x", (int)ch); System.out.println(s); } } } } |
DASH_PUNCTUATION
DASH_PUNCTUATION – Categoria geral “Pd” na especificação Unicode.
public static final byte DASH_PUNCTUATION – Categoria geral “Pd” na especificação Unicode.
Esse método existe desde a versão 1.1.
Veja também:
Fonte do código: http://www.java2s.com/Tutorials/Java/java.lang/Character/0120__Character.DASH_PUNCTUATION.htm
1 2 3 4 5 6 7 8 9 10 |
<strong>public</strong> <strong>class</strong> Main{ <strong>public</strong> <strong>static</strong> <strong>void</strong> main(String[] args) { <strong>for</strong>(<strong>char</strong> ch = Character.MIN_VALUE;ch<Character.MAX_VALUE;ch++){ <strong>if</strong>(Character.DASH_PUNCTUATION == Character.getType(ch)){ String s = String.format (<strong>"\\u%04x"</strong>, (<strong>int</strong>)ch); System.out.println(s);/*from ww w . j a v a 2 s .c om*/ } } } } |
DECIMAL_DIGIT_NUMBER
DECIMAL_DIGIT_NUMBER – Categoria geral “Nd” na especificação Unicode.
public static final byte DECIMAL_DIGIT_NUMBER – Categoria geral “Nd” na especificação Unicode.
Esse método existe desde a versão 1.1.
Veja também:
Fonte: https://docs.oracle.com/en/java/javase/14/docs/api/java.base/java/lang/Character.html
Deixe um comentário